SECTION A
1.Short answer (explain High Level Language, OOP, Class, Object, Abstraction, Graphic (AWT and Swing))
2.Show output (selection, looping etc)
example:: Show output of the following java code :-
int x,y;
x=7,y=5;
if(a>b)
System.out.println (“Yes”);
else
System.out.println (“No”);
3.Draw flowchart of a given code
SECTION B
1.Show output and draw UML diagram for a given code.
2.Write a simple java program (ex::input a value in fahrenheit, convert to celcius. Read 50 integer and print in reverse order)
SECTION C
Write a class based on given data or instruction. (same question given during lab session)
12/17/2013
12/09/2013
BCS2233 OOP: Quiz 4
Name:_______________________
Matric:_______________________
Course:_______________________
CHAPTER 12
1. Explain the difference between AWT GUI components, such as java.awt.Button, and Swing components, such as javax.swing.JButton.
2. Show the output of the following java code:
import javax.swing.*;
public class Test {
public static void main(String[] args) {
JButton jbtOK = new JButton("OK");
System.out.println(jbtOK.isVisible());
JFrame frame = new JFrame();
System.out.println(frame.isVisible());
}
}
Matric:_______________________
Course:_______________________
CHAPTER 12
1. Explain the difference between AWT GUI components, such as java.awt.Button, and Swing components, such as javax.swing.JButton.
2. Show the output of the following java code:
import javax.swing.*;
public class Test {
public static void main(String[] args) {
JButton jbtOK = new JButton("OK");
System.out.println(jbtOK.isVisible());
JFrame frame = new JFrame();
System.out.println(frame.isVisible());
}
}
12/01/2013
BCS2233 OOP: Quiz 3
Name:_______________________
Matric:_______________________
Course:_______________________
A. TRUE/ FALSE (5 Marks)
1. You can derive a new class from an existing class. This is called as class inheritance. (T/F)
2. The new class (derived class ) is called a superclass. The existing class is called child class.(T/F)
3. Constructors are a special kind of methods that are invoked to construct objects(T/F)
4. To override a method, the method must be defined in the derived class using the same signature as in its existing class.(T/F)
5. All method including a private method can be overridden.(T/F)
B. Design a class named person and its two subclasses named student and employee. A person has a name, address, phone, and email address. A student has a status. An employee has an department, salary and date hired.
Draw the UML diagram for the classes.
(10 Marks)
* untuk soalan B. 1. Bina Class (tak perlu buat program penuh) 2. Lukis UML.
pls submit before 5:00 pm.
Matric:_______________________
Course:_______________________
A. TRUE/ FALSE (5 Marks)
1. You can derive a new class from an existing class. This is called as class inheritance. (T/F)
2. The new class (derived class ) is called a superclass. The existing class is called child class.(T/F)
3. Constructors are a special kind of methods that are invoked to construct objects(T/F)
4. To override a method, the method must be defined in the derived class using the same signature as in its existing class.(T/F)
5. All method including a private method can be overridden.(T/F)
B. Design a class named person and its two subclasses named student and employee. A person has a name, address, phone, and email address. A student has a status. An employee has an department, salary and date hired.
Draw the UML diagram for the classes.
(10 Marks)
* untuk soalan B. 1. Bina Class (tak perlu buat program penuh) 2. Lukis UML.
pls submit before 5:00 pm.
11/24/2013
BCS2233 OOP: Quiz 2
1. TRUE / FLASE (5 Marks)
2. Consider the following java code. Draw the UML diagram for the class cubee.(5 Marks)
import java.util.Scanner;
public class class1_triangle {
public static void main(String[] args) {
cubee mycube= new cubee();
mycube.inputdata();
System.out.println("Area of a cube is " + mycube.get_area());
System.out.println("Perimeter of a cube is " + mycube.get_parameter());
}
}
class cubee
{
int width;
void inputdata()
{
System.out.println("Please enter width of a cube ");
Scanner input = new Scanner(System.in);
width = input.nextInt();
}
double get_area()
{
return (width * width*width);
}
double get_parameter()
{
return width*12;
}
}
2. Consider the following java code. Draw the UML diagram for the class cubee.(5 Marks)
import java.util.Scanner;
public class class1_triangle {
public static void main(String[] args) {
cubee mycube= new cubee();
mycube.inputdata();
System.out.println("Area of a cube is " + mycube.get_area());
System.out.println("Perimeter of a cube is " + mycube.get_parameter());
}
}
class cubee
{
int width;
void inputdata()
{
System.out.println("Please enter width of a cube ");
Scanner input = new Scanner(System.in);
width = input.nextInt();
}
double get_area()
{
return (width * width*width);
}
double get_parameter()
{
return width*12;
}
}
11/18/2013
BCS2233 OOP: Assignment 2 & 3
ASSIGNMENT 2
1. Write method headers for the following methods:
a. Computing a sales commission, given the sales amount and the commission rate.
b. Printing the calendar for a month, given the month and year.
c. Computing a square root.
d. Testing whether a number is even, and returning true if it is.
e. Printing a message a specified number of times.
f. Computing the monthly payment, given the loan amount, number of years, and annual interest rate.
2. (Analyzing input) Write a program that reads ten numbers, computes their average, and finds out how many numbers are above the average.
3. (Reversing the numbers entered) Write a program that reads ten integers and displays them in the reverse of the order in which they were read.
ASSIGNMENT 3
1. Define the following terms:-
a. class
b. object
c. data
d. properties
e. behaviour
f. method
g. constructor
h. destructor
2. (The Rectangle class) Design a class named Rectangle to represent a rectangle. The class contains:
Two double data fields named width and height that specify the width and height of the rectangle. The default values are 1 for both width and height.
A string data field named color that specifies the color of a rectangle. Hypothetically, assume that all rectangles have the same color. The default color is white.
A no-arg constructor that creates a default rectangle.
A constructor that creates a rectangle with the specified width and height.
The accessor and mutator methods for all three data fields.
A method named getArea() that returns the area of this rectangle.
A method named getPerimeter() that returns the perimeter.
Draw the UML diagram for the class. Implement the class. Write a test program that creates two Rectangle objects. Assign width 4 and height 40 to the first object and width 3.5 and height 35.9 to the second object. Assign color red to all Rectangle objects. Display the properties of both objects and find their areas and perimeters.
1. Write method headers for the following methods:
a. Computing a sales commission, given the sales amount and the commission rate.
b. Printing the calendar for a month, given the month and year.
c. Computing a square root.
d. Testing whether a number is even, and returning true if it is.
e. Printing a message a specified number of times.
f. Computing the monthly payment, given the loan amount, number of years, and annual interest rate.
2. (Analyzing input) Write a program that reads ten numbers, computes their average, and finds out how many numbers are above the average.
3. (Reversing the numbers entered) Write a program that reads ten integers and displays them in the reverse of the order in which they were read.
ASSIGNMENT 3
1. Define the following terms:-
a. class
b. object
c. data
d. properties
e. behaviour
f. method
g. constructor
h. destructor
2. (The Rectangle class) Design a class named Rectangle to represent a rectangle. The class contains:
Two double data fields named width and height that specify the width and height of the rectangle. The default values are 1 for both width and height.
A string data field named color that specifies the color of a rectangle. Hypothetically, assume that all rectangles have the same color. The default color is white.
A no-arg constructor that creates a default rectangle.
A constructor that creates a rectangle with the specified width and height.
The accessor and mutator methods for all three data fields.
A method named getArea() that returns the area of this rectangle.
A method named getPerimeter() that returns the perimeter.
Draw the UML diagram for the class. Implement the class. Write a test program that creates two Rectangle objects. Assign width 4 and height 40 to the first object and width 3.5 and height 35.9 to the second object. Assign color red to all Rectangle objects. Display the properties of both objects and find their areas and perimeters.
11/11/2013
BCS2233 OOP : LAB WEEK 10
Read ten numbers, compute their average, and find out how many numbers are above AND BELOW the average.
refer to powerpoint slide page 3 on chapter 6 for the solution.
refer to powerpoint slide page 3 on chapter 6 for the solution.
11/07/2013
DCT1043 COA. QUIZ1, ASSIGNMENT1 dan TEST1
Markah untuk QUIZ1, ASSIGNMENT 1 dan TEST 1.
Markah QUIZ 1 di ambil daripada markah tertinggi antara Quiz 1 dan Exercise1 yang di berikan.
Pelajar yang belum mengambil test 1 dan belum menghantar assignment 1 sila berbuat demikian segera.
No. | Matric No. | Course | Name | Quiz 1 (100%) | Test (100%) | asgmt 1 (100%) |
1 | 12A13005 | DCIT | HANIF ANNAIM BIN ABDILLAH | 75.0 | 33.3 | 71.4 |
2 | 12A13014 | DCIT | MOHD FAIZ BIN JAMALUDIN | 83.3 | 68.6 | 71.4 |
3 | 13A09060 | DCIT | NURUL AZIANA BT JAAFAR ALBAR | 50.0 | 45.1 | 85.7 |
4 | 13A12054 | DCIT | PUTERA NURZULHARRIQ FITRI BIN ZAWAWI | 0.0 | #VALUE! | #VALUE! |
5 | 13A13001 | DCIT | ABU UBAIDAH BIN HANAFI | 66.7 | 51.0 | 68.6 |
6 | 13A13002 | DCIT | AFDILLA BINTI ROZMAN | 72.2 | 54.9 | 85.7 |
7 | 13A13005 | DCIT | AHMAD HAEKAI BIN MANAP | 66.7 | 27.5 | 62.9 |
8 | 13a13007 | DCIT | AHMAD RASHDAN BIN AHMAD AFFANDI | 72.2 | 78.4 | 65.7 |
9 | 13A13008 | DCIT | AIDA YUSMARLINA BINTI YUSOP | 79.2 | 60.8 | 88.6 |
10 | 13A13010 | DCIT | ARIFF BIN ABD KARIM ISHIGAKI | 79.2 | 76.5 | #VALUE! |
11 | 13A13016 | DCIT | MOHAMAD SYAFIQ IZUDEN BIN HASSAN | 0.0 | 58.8 | #VALUE! |
12 | 13a13017 | DCIT | MOHAMMADI ALMEE BIN RAZAK | 72.2 | 52.9 | 77.1 |
13 | 13A13019 | DCIT | MOHD FARIS ASYRAF BIN MOHD ISMU | 75.0 | 62.7 | 65.7 |
14 | 13A13020 | DCIT | MUHAMAD ASYRAFF BIN ADNAN | 77.8 | #VALUE! | 62.9 |
15 | 13A13022 | DCIT | MUHAMAD FARED THALABI BIN BAHARUN | 88.9 | 80.4 | 60.0 |
16 | 13A13023 | DCIT | MUHAMAD NAZIRUL HAFIZIE B ABDUL WAHID | 72.2 | 39.2 | #VALUE! |
17 | 13A13025 | DCIT | MUHAMAD ZURIA SHAFIQ ZAINUDDIN BIN MUSA | 88.9 | 49.0 | 65.7 |
18 | 13A13028 | DCIT | MUHAMMAD AMIRUL AMRI BIN ABDUL RAZAK | 50.0 | #VALUE! | #VALUE! |
19 | 13A13031 | DCIT | MUHAMMAD FIRDAUS BIN CHE AWANG | 75.0 | 49.0 | 65.7 |
20 | 13A13032 | DCIT | MUHAMMAD HAFIZ SHARUL BIN WAHED | 66.7 | 37.3 | 65.7 |
21 | 13a13034 | DCIT | MUHAMMAD MUHAIMIN BIN MOHD SAIDI | 87.5 | 64.7 | 60.0 |
22 | 13A13035 | DCIT | MUHAMMAD SYAFIQ SAIFUDDIN BIN STAPA | 75.0 | 58.8 | 48.6 |
23 | 13a13037 | DCIT | MUHD HAFIZ BIN ROSLI | 0.0 | #VALUE! | #VALUE! |
24 | 13a13041 | DCIT | NOORAINI BINTI HUSSAIN | 54.2 | 52.9 | #VALUE! |
25 | 13a13044 | DCIT | NOR HAZIEM BIN SHAMSUDIN | 62.5 | 86.3 | 65.7 |
26 | 13a13045 | DCIT | NORHAZIRA BINTI ZAKARIA | 72.2 | 33.3 | #VALUE! |
27 | 13a13046 | DCIT | NUR HAMALINA BINTI AL-AZMI | 70.8 | 49.0 | 85.7 |
28 | 13a13050 | DCIT | NUR SYAHIDAH BINTI MASRUDDIN | 72.2 | 62.7 | 77.1 |
29 | 13A13053 | DCIT | NURUL ADIELLA BINTI MOHD FANDI | 61.1 | 58.8 | 74.3 |
30 | 13a13055 | DCIT | NURUL HAZWANI BINTI HARUN | 77.8 | 39.2 | 77.1 |
31 | 13A13056 | DCIT | NURUL IBTISAM BT CHE KU MAH HUSSAIN | 41.7 | #VALUE! | #VALUE! |
32 | 13a13060 | DCIT | RUSHAZLLAN BIN MOHD NOOR | 66.7 | 35.3 | 65.7 |
33 | 13a13063 | DCIT | SHAZALIANA BT ROSLI | 66.7 | 54.9 | 74.3 |
34 | 13A13065 | DCIT | TUAN MOHAMAD SADRUDDIN BIN TUAN ISMAIL | 0.0 | #VALUE! | #VALUE! |
35 | 13a13068 | DCIT | WAN MUHAMMAD IRWAN SYAFIKI BIN WAN MOHD NOR | 83.3 | 94.1 | 65.7 |
36 | 13A13069 | DCIT | NABILA BINTI MAHUSAIN | 77.8 | 58.8 | 85.7 |
37 | 13A13070 | DCIT | MOHD FARIDUDDIN RIDHWAH BIN MAZLAN | 77.8 | 82.4 | 65.7 |
38 | 13A13071 | DCIT | ABDUL HAFIZ BIN ABDULLAH | 79.2 | #VALUE! | #VALUE! |
39 | 13C19022 | DCIT | NUR AFIQAH FARAHIN BT MOHD ASRI | 72.2 | 39.2 | 85.7 |
40 | 13A12054 | DCIT | PUTERA NURZULHARRIQ FITRI BIN ZAWAWI | 50.0 | 25.5 | 65.7 |
Markah QUIZ 1 di ambil daripada markah tertinggi antara Quiz 1 dan Exercise1 yang di berikan.
Pelajar yang belum mengambil test 1 dan belum menghantar assignment 1 sila berbuat demikian segera.
11/05/2013
DCT1083: Markah test 1 dan status asgmt 2 dan asgmt 3
Assalamualaikum. Berikut adalah markah untuk test 1. Oleh kerana saya masih belum habis tanda asgmt 1 dan asgmt 2 maka saya tandakan H untuk maksud Hantar. Yang belum hantar- sila berbuat demikian. Jika lewat penalti (tolak markah) akan di kenakan.
Saya akan update dari masa ke semasa. Terima Kasih.
No. | Matric No. | Course | Name | TEST 1 (100) | asgmt2 | asgmt3 |
1 | 13A05001 | NT | ABDUL HAKIM ULWAN BIN AZRI | 33.1 | H | |
2 | 13A05003 | NT | ABDUL ZULHILMI BADARUDDIN BIN ABD PAHMY | 0.0 | ||
3 | 13A05004 | NT | AHMAD ALIF ARSYAD BIN ZANIL | 32.3 | ||
4 | 13A05005 | NT | AHMAD JANEY BIN AB RAZAK | 27.7 | H | |
5 | 13A05007 | NT | AMIR HAMZAH BIN HARUN | 38.5 | H | |
6 | 13A05008 | NT | AMIRUL HAKIMI BIN HAMDAN | 35.4 | H | |
7 | 13A05009 | NT | AMIRULL MUSTAQIM BIN MOHAMMAD | 77.7 | H | |
8 | 13A05010 | NT | AZMIL BIN ANUA | 30.8 | H | |
9 | 13A05011 | NT | CHE KU ATYRAH FARHANA BT CHE KU MUSA | 38.5 | H | H |
10 | 13A05012 | NT | CHE MUHAMAD AIZAT IZUDDIN BIN CHE PAUZI | 54.6 | H | |
11 | 13A05013 | NT | EMMIN EFFENDI B ROSLI | 26.2 | H | |
12 | 13A05014 | NT | ENGKU MUHAMMAD FIRDAUS BIN ENGKU YUSOFF | 27.7 | H | |
13 | 13A05016 | NT | FATIN NADHIRAH BINTI MD ZUBIR | 27.7 | H | H |
14 | 13A05017 | NT | MAISARAH SAMIHAH BINTI MOHD SETAPAI | 31.5 | H | H |
15 | 13A05018 | NT | MOHAMAD AMIN BIN AHMAD | 0.0 | ||
16 | 13A05021 | NT | MOHAMMAD IHSAN FAKHRI B KADRIS | 32.3 | ||
17 | 13A05022 | NT | MOHD AMIRUL ZAABA B. MOHD RANI | 36.9 | H | |
18 | 13A05024 | NT | MOHD KHAIRI AZIZI BIN MOHD SIDEK | 0.0 | ||
19 | 13A05025 | NT | MOHD NAZRUL HISYAMMUDIN BIN JAFRYDIN | 56.2 | H | |
20 | 13A05026 | NT | MOHD NUR FIKRI ANIFF B MAT ANAH | 25.4 | H | |
21 | 13A05027 | NT | MUHAMAD FADILAH BIN MOHAMMAD | 66.2 | H | |
22 | 13A05028 | NT | MUHAMMAD AIAN SHAKIR BIN MOHD KAMAL ARIFFIN | #VALUE! | ||
23 | 13A05029 | NT | MUHAMMAD ALLIF BIN MOHD RADZIF | 37.7 | ||
24 | 13A05030 | NT | MUHAMMAD AMIR ASYRAF B ABDULLAH | 26.2 | ||
25 | 13A05032 | NT | MUHAMMAD AMIRUL ASYRAF BIN ABDUL RAHMAN @ ZAKARIA | 59.2 | H | |
26 | 13A05034 | NT | MUHAMMAD ASYRAF BIN AZMAN | 0.0 | ||
27 | 13A05035 | NT | MUHAMMAD ASYRAF BIN MAT NOR | 90.0 | H | |
28 | 13A05036 | NT | MUHAMMAD FAKHRI BIN ABDUL SHUKOR | 32.3 | ||
29 | 13A05037 | NT | MUHAMMAD FIRDAUS BIN MOHD ISMAON | 29.2 | H | |
30 | 13A05040 | NT | MUHAMMAD SYAZWAN FADHLI BIN ROSDI | 27.7 | H | |
31 | 13A05041 | NT | MUHAMMAD ZULHAKIMI AMRY BIN ZULKEFLI | 47.7 | ||
32 | 13A05042 | NT | NAJIHAH BINTI MASUMI | 36.2 | H | H |
33 | 13A05043 | NT | NIK AHMAD FARIS DANIAL BIN NIK M GHAZALI | 0.0 | ||
34 | 13A05044 | NT | NOOR IDAYU BINTI SULAIMAN | 26.2 | H | H |
35 | 13A05049 | NT | NUR DHIYA ALYANI BINTI RADUAN | 86.2 | H | H |
36 | 13A05051 | NT | NUR HAFIZAH BINTI MOHAMAD | 56.2 | H | H |
37 | 13A05054 | NT | NURHAQQI BIN NUZUAMRY | 0.0 | ||
38 | 13A05055 | NT | NURSHAMIM SYAFIQAH BINTI CHE MOHD SUPARDI | 40.0 | H | H |
39 | 13A05056 | NT | NURUL AFIQAH BINTI SAHARI | 19.2 | H | H |
40 | 13A05057 | NT | NURUL AMIRAH BT ZULBAHARI | 73.1 | H | H |
41 | 13A05058 | NT | NURUL ARIANIE BINTI ALI | 19.2 | H | H |
42 | 13A05059 | NT | NURUL IRZUWANI BT ROSELAN | 0.0 | ||
43 | 13A05060 | NT | NURUL NAJIHAH BINTI MOHAMED | 25.4 | H | H |
44 | 13A05061 | NT | NURUL NURFATIHAH BT MOHD DAROS | 0.0 | ||
45 | 13A05064 | NT | NURYUSAIRAH BT ZAINORDIN | 0.0 | ||
46 | 13A05065 | NT | RABBIATUL ADDAWIYYAH BT MOHD SAYUTI | 33.1 | ||
47 | 13A05066 | NT | RAMADHAN AL- MUBARAQ BIN MOHAMAD | 30.0 | ||
48 | 13A05067 | NT | SHARMIN FITRI BIN SHUHAIMI | 29.2 | H | |
49 | 13A05068 | NT | SITI HAJAR SHAKIRAH BINTI NOR ASHARI | 44.6 | ||
50 | 13A05069 | NT | TUAN MOHAMAD FAKHRURAZIE BIN TUAN ZAINAL ADLI | 86.2 | H | |
51 | 13A05071 | NT | WAN AHMAD FIRDAOUS BIN WAN AHMAD SAZLI | 33.1 | H | |
52 | 13A05075 | NT | AHMAD ZURAIRI `ANIF BIN AMRI | 29.2 | H |
Subscribe to:
Posts (Atom)
Cara download Installer windows 10 dalam format ISO
1. Jika anda bercadang untuk download windows 10 melalui website rasmi windows - pilihan untuk download dalam format ISO tidak di berikan. ...
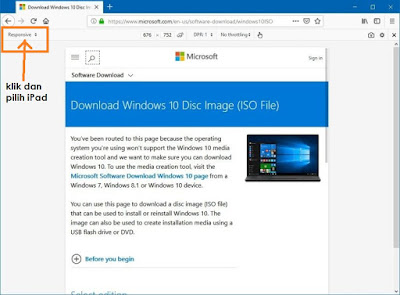
-
ANSWERS TO CHAPTER EXERCISES (CHAPTER 8) Review Questions 1. List and describe various types of output, including technolog...
-
Based on real life scenario on any local company/ organization which you are very familiar, define the problem that could be addressed thr...
-
A. Response to the following statements by circling either T (TRUE) or F (FALSE). [10M] I. 1. Java enables users...