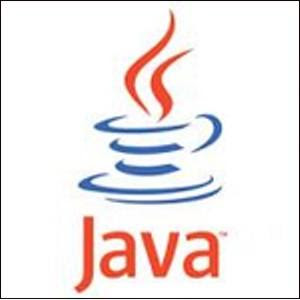
Write method headers for the following methods:
1. Computing a sales commission, given the sales amount and the commission rate.
2. Testing whether a number is even, and returning true if it is.
3. Printing a message a specified number of times.
4. Computing the monthly payment, given the loan amount, number of years, and annual interest rate.
/**
* @(#)TestMax.java
*
* TestMax application
*
* @author
* @version 1.00 2010/8/23
*/
public class TestMax {
public static void main(String[] args) {
int i = 5;
int j = 2;
int k = max(i, j);
System.out.println("The maximum between " + i +
" and " + j + " is " + k);
System.out.println(comm(100,20)); // question 1
System.out.println(TestEven(5));// question 2
System.out.println(MP(43000,7,3.88)); // question 4
}
/** Return the max between two numbers */
public static int max(int num1, int num2) {
int result;
if (num1 > num2)
result = num1;
else
result = num2;
return result;
}
/** Comm -Return commission of a given amount sales and % commission rate */
public static double comm(double amount,double cr)
{
return (amount * cr/100);
}
public static boolean TestEven(int N)
{
if((N%2)==0)
return true;
else
return false;
}
public static double MP(double loan, int year, double IR)
{
double Total_interest_rate = year*IR;
double Total_Payment = (loan * Total_interest_rate /100)+ loan;
return Total_Payment /year/12;
}
}