Give both Structured and Object Oriented solution for the following problems.
1. Write a java program that reads the length and height of a rectangle and computes its area and parameter using the following formulas:-
Area = length x height
Perimeter = 2 x (length + height)
Structured: https://drive.google.com/file/d/0BxDLK8tlx2V1dEo0MGRRY3FLcmM/view?usp=sharing
2. Write a java program that reads the height of a cube and computes its area and parameter using the following formulas:-
Area = height2
Perimeter = 4 x height
Structured: https://drive.google.com/file/d/0BxDLK8tlx2V1MzM4SERjQkctLUk/view?usp=sharing
3. Write a program that reads in the radius and length of a cylinder and computes its volume using the following formulas:
Area = radius * radius * 3.14
Volume = area * length
4/24/2018
4/22/2018
DCT2023 DS & FP. Lab Linked Lists. (#2)
1. Write a program in C++ to insert a new node at the
beginning of a Singly Linked List.
Test Data :
Input the number of nodes : 3
Input data for node 1 : 5
Input data for node 2 : 6
Input data for node 3 : 7
Expected Output :
Data entered in the list :
Data = 5
Data = 6
Data = 7
Solution (you may use your own solution)
2. Write a program in C++ to create a singly linked list of n nodes and count the number of nodes.
Test Data :
Input the number of nodes : 3
Input data for node 1 : 5
Input data for node 2 : 6
Input data for node 3 : 7
Expected Output :
Data entered in the list are :
Data = 5
Data = 6
Data = 7
Total number of nodes = 3
3. Write a program in C++ to insert a new node at the end of a Singly Linked List.
Test Data and Expected Output :
Test Data :
Input the number of nodes : 3
Input data for node 1 : 5
Input data for node 2 : 6
Input data for node 3 : 7
Expected Output :
Data entered in the list :
Data = 5
Data = 6
Data = 7
Solution (you may use your own solution)
2. Write a program in C++ to create a singly linked list of n nodes and count the number of nodes.
Test Data :
Input the number of nodes : 3
Input data for node 1 : 5
Input data for node 2 : 6
Input data for node 3 : 7
Expected Output :
Data entered in the list are :
Data = 5
Data = 6
Data = 7
Total number of nodes = 3
3. Write a program in C++ to insert a new node at the end of a Singly Linked List.
Test Data and Expected Output :
Input the number of nodes : 3 Input data for node 1 : 5 Input data for node 2 : 6 Input data for node 3 : 7 Data entered in the list are : Data = 5 Data = 6 Data = 7 Input data to insert at the end of the list : 8 Data, after inserted in the list are : Data = 5 Data = 6 Data = 7
Data = 8
4. Write a program in C++ to insert a new node at the middle of Singly Linked List. Test Data and Expected Output : Input the number of nodes (3 or more) : 4 Input data for node 1 : 1 Input data for node 2 : 2 Input data for node 3 : 3 Input data for node 4 : 4 Data entered in the list are : Data = 1 Data = 2 Data = 3 Data = 4 Input data to insert in the middle of the list : 5 Input the position to insert new node : 3 Insertion completed successfully. The new list are : Data = 1 Data = 2 Data = 5 Data = 3 Data = 4
5. Write a program in C++ to delete first node of Singly Linked List. Test Data : Input the number of nodes : 3 Input data for node 1 : 2 Input data for node 2 : 3 Input data for node 3 : 4 Expected Output : Data entered in the list are : Data = 2 Data = 3 Data = 4 Data of node 1 which is being deleted is : 2 Data, after deletion of first node : Data = 3 Data = 4
6. Write a program in C++ to delete a node from the middle of Singly Linked List. Test Data and Expected Output : Input the number of nodes : 3 Input data for node 1 : 2 Input data for node 2 : 5 Input data for node 3 : 8 Data entered in the list are : Data = 2 Data = 5 Data = 8 Input the position of node to delete : 2 Deletion completed successfully. The new list are : Data = 2 Data = 8
7. Write a program in C++ to delete the last node of Singly Linked List. Test Data : Input the number of nodes : 3 Input data for node 1 : 1 Input data for node 2 : 2 Input data for node 3 : 3 Expected Output : Data entered in the list are : Data = 1 Data = 2 Data = 3 The new list after deletion the last node are : Data = 1 Data = 2
8. Write a program in C++ to search an existing element in a singly linked list. Test Data and Expected Output : Input the number of nodes : 3 Input data for node 1 : 2 Input data for node 2 : 5 Input data for node 3 : 8 Data entered in the list are : Data = 2 Data = 5 Data = 8 Input the element to be searched : 5 Element found at node 2
4/17/2018
BCS2233 OOP. Method.
By implementing functions (also called methods) in a program, programmer
reduces coding time and debugging time, thereby reducing the overall
development time.
Consider the following Java program. Create and use method where necessary.
Consider the following Java program. Create and use method where necessary.
//program 1
import java.util.Scanner;
public class tambahNombor {
public static void main(String[] args) {
int
N1,N2,hasiltambah;
Scanner
input= new Scanner(System.in);
System.out.println("Sila
masukkan dua nombor");
N1=
input.nextInt();
N2=
input.nextInt();
hasiltambah=N1+N2;
System.out.println("Hasil
tambah dua nombor ialah "+hasiltambah);
System.out.println("Sila
masukkan dua nombor");
N1=
input.nextInt();
N2=
input.nextInt();
hasiltambah=N1+N2;
System.out.println("Hasil
tambah dua nombor ialah "+hasiltambah);
System.out.println("Sila
masukkan dua nombor");
N1=
input.nextInt();
N2=
input.nextInt();
hasiltambah=N1+N2;
System.out.println("Hasil
tambah dua nombor ialah "+hasiltambah);
System.out.println("Sila
masukkan dua nombor");
N1=
input.nextInt();
N2=
input.nextInt();
hasiltambah=N1+N2;
System.out.println("Hasil
tambah dua nombor ialah "+hasiltambah);
}
}
//program 2
import
java.util.Scanner;
public class sumInteger {
public
static void main(String[] args) {
int N1,N2,sum,count;
Scanner input = new
Scanner(System.in);
System.out.println("Sila
masukkan dua nombor!");
N1= input.nextInt();
N2= input.nextInt();
sum=0;
for(count=N1;count<=N2;count++)
{
sum=sum+count;
}
System.out.println("Hasil tambah "+N1+" hingga
"+N2+" ialah " + sum);
System.out.println("Sila masukkan dua nombor ");
N1= input.nextInt();
N2= input.nextInt();
sum=0;
for(count=N1;count<=N2;count++)
{
sum=sum+count;
}
System.out.println("Hasil tambah "+N1+" hingga
"+N2+" ialah " + sum);
System.out.println("Sila masukkan dua nombor!");
N1= input.nextInt();
N2= input.nextInt();
sum=0;
for(count=N1;count<=N2;count++)
{
sum=sum+count;
}
System.out.println("Hasil tambah "+N1+" hingga
"+N2+" ialah " + sum);
}
}
//program 3
import java.util.Scanner;
public class bmi {
public static void main(String[] args) {
double
berat,tinggi,BMI;
String
status;
Scanner
input = new Scanner(System.in);
System.out.println("Sila
masukkan berat (KG) dan tinggi (Meter)");
berat
= input.nextDouble();
tinggi
= input.nextDouble();
BMI=berat/(tinggi*tinggi);
if(BMI<18 .5="" p="">
System.out.println("underweight");
else
if((BMI>=18.5)&&(BMI<=24.9))
System.out.println("healthy");
else
if((BMI>=25)&&(BMI<=29.9))
System.out.println("overweight");
else
if (BMI>=30)
System.out.println("obes");
System.out.println("Sila
masukkan berat (KG) dan tinggi (Meter)");
berat
= input.nextDouble();
tinggi
= input.nextDouble();
BMI=berat/(tinggi*tinggi);
if(BMI<18 .5="" p="">
18>
System.out.println("underweight");
else
if((BMI>=18.5)&&(BMI<=24.9))
System.out.println("healthy");
else
if((BMI>=25)&&(BMI<=29.9))
System.out.println("overweight");
else
if (BMI>=30)
System.out.println("obes");
System.out.println("Sila
masukkan berat (KG) dan tinggi (Meter)");
berat
= input.nextDouble();
tinggi
= input.nextDouble();
BMI=berat/(tinggi*tinggi);
if(BMI<18 .5="" p="">
18>
18>
System.out.println("underweight");
else
if((BMI>=18.5)&&(BMI<=24.9))
System.out.println("healthy");
else
if((BMI>=25)&&(BMI<=29.9))
System.out.println("overweight");
else
if (BMI>=30)
System.out.println("obes");
}
}
//program 4
import java.util.Scanner;
public class ulangPesanan {
public static void main(String[] args) {
Scanner
input = new Scanner(System.in);
String
pesanan;
int
i,jumlahUlangan;
System.out.println("Sila
masukkan Pesanan :");
pesanan
= input.nextLine();
System.out.println("Berapa
kali nak ulang ? :");
jumlahUlangan
= input.nextInt();
for (i=1;i<=jumlahUlangan;i++)
{
System.out.println(pesanan);
}
System.out.println("Sila masukkan
Pesanan :");
pesanan
= input.nextLine();
System.out.println("Berapa
kali nak ulang ? :");
jumlahUlangan
= input.nextInt();
for (i=1;i<=jumlahUlangan;i++)
{
System.out.println(pesanan);
}
System.out.println("Sila masukkan
Pesanan :");
pesanan
= input.nextLine();
System.out.println("Berapa
kali nak ulang ? :");
jumlahUlangan
= input.nextInt();
for (i=1;i<=jumlahUlangan;i++)
{
System.out.println(pesanan);
}
}
}
18>
Subscribe to:
Posts (Atom)
Cara download Installer windows 10 dalam format ISO
1. Jika anda bercadang untuk download windows 10 melalui website rasmi windows - pilihan untuk download dalam format ISO tidak di berikan. ...
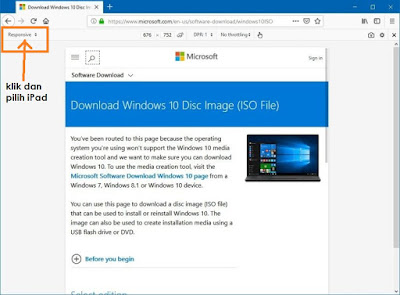
-
ANSWERS TO CHAPTER EXERCISES (CHAPTER 8) Review Questions 1. List and describe various types of output, including technolog...
-
Based on real life scenario on any local company/ organization which you are very familiar, define the problem that could be addressed thr...
-
A. Response to the following statements by circling either T (TRUE) or F (FALSE). [10M] I. 1. Java enables users...