Programming Exercise 1.
(please provide flowchart and java program for each question. You can create flowchart online at https://www.draw.io/)
1. (Calculate body mass index (BMI) ) Write a program that reads a Weight and Height, then calcculate BMI and displays the result . The formula
for the BMI is as follows:
BMI = Weight / (Height x Height);
2. (Converting Fahrenheit to Celsius) Write a program that reads a
Fahrenheit degree, then converts it
to Celsius and displays the result . The formula
for the conversion is as follows:
celsius = (5/9) * (fahrenheit - 32)
Hint
In Java, 5 / 9 is 0, so you need to write 5.0 / 9 in the program to obtain the correct result.
3. (Computing the volume of a cylinder) Write a program that reads in the
radius and length of a cylinder and computes its volume using the
following formulas:
area = radius * radius * pi
volume = area * length
(pi = 3.14)
Solution
Question 1.
import java.util.Scanner;
public class hello1 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
// Prompt the user to enter weight in KG
System.out.print("Enter weight in Kilogram: ");
double weight = input.nextDouble();
// Prompt the user to enter height in Meters
System.out.print("Enter height in Meters: ");
double height = input.nextDouble();
// Compute BMI
double bmi = weight / (height * height);
// Display result
System.out.println("Your BMI is " + bmi);
}
}
Subscribe to:
Post Comments (Atom)
Cara download Installer windows 10 dalam format ISO
1. Jika anda bercadang untuk download windows 10 melalui website rasmi windows - pilihan untuk download dalam format ISO tidak di berikan. ...
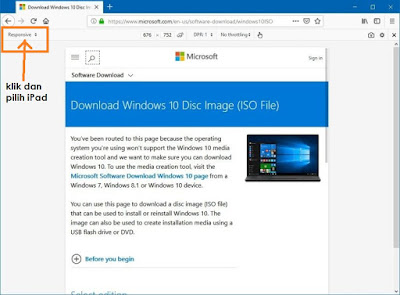
-
ANSWERS TO CHAPTER EXERCISES (CHAPTER 8) Review Questions 1. List and describe various types of output, including technolog...
-
Based on real life scenario on any local company/ organization which you are very familiar, define the problem that could be addressed thr...
-
Latihan MS Word dengan elemen-elemen:- image, word art, table, shape dan word formatting (font,size,bold, italic,underline)
No comments:
Post a Comment